Preload Images And Other Assets With JavaScript
July 5, 2018 by Andreas Wik
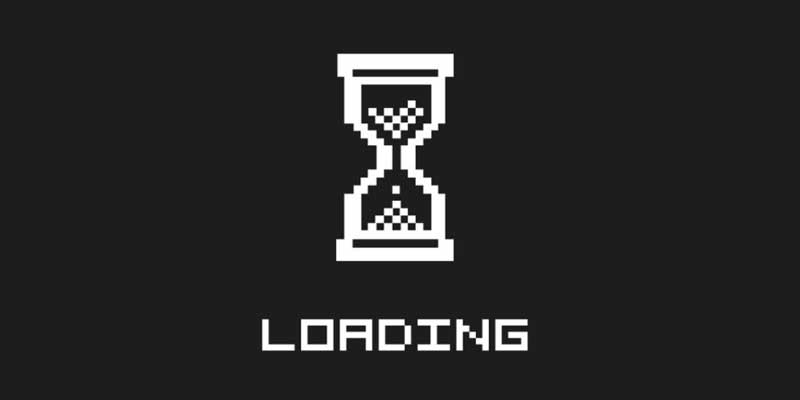
If you’re building, say, a game, or maybe a beautiful website with a lot of heavy graphics, you might want to make sure everything is loaded before you present it.
With Preload JS we can do exactly this. Let’s dive right in.
First of all, include Preload JS. I’ll grab it from their CDN:
<script src="https://code.createjs.com/preloadjs-0.6.2.min.js"></script>
Set up a button which will call our loadAssets function and a div which will use to show the progress:
<div id="preload-container">
<button onclick="loadAssets();">START LOADING</button>
</div>
Time for some JS. Let’s create a function that we can call when we want to start loading our assets:
const loadAssets = () => {
// Start loading assets
document.getElementById('preload-container').innerHTML = '<h1>LOADING... <span id="loading-percent">' + 0 + '</span>%</h1>';
};
Inside of this function, first create a load queue:
const queue = new createjs.LoadQueue();
We also need to create a loadManifest which contains the URL’s for all the assets we want to load:
queue.loadManifest([
{id: 'img1', src: 'https://images.unsplash.com/photo-1530379062299-a950185fdf6c?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=191d8c6bd6adc2e6b889bcde1731313c&auto=format&fit=crop&w=3000&q=100'},
{id: 'img2', src: 'https://images.unsplash.com/photo-1530758984765-9fa189425c28?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=f672f00aa31f1d63bf13e3af6d0e7aa8&auto=format&fit=crop&w=2500&q=100'},
{id: 'img3', src: 'https://images.unsplash.com/photo-1530750463537-af1c747d8d73?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=7e6252a08cf41d9b0ed59f08b3456854&auto=format&fit=crop&w=3000&q=100'}
]);
Let’s also set up a couple of event handlers. In this case we’ll call our function handleProgress which will be run as soon as there is some progress loading assets, and handleComplete which will be called once everything is done loading.
queue.on("progress", handleProgress, this);
queue.on("complete", handleComplete, this);
The last line we need in our loadAssets function is this, which is what starts the actual loading:
queue.load();
loadAssets final:
const loadAssets = () => {
document.getElementById('preload-container').innerHTML = '<h1>LOADING... <span id="loading-percent">' + 0 + '</span>%</h1>';
const queue = new createjs.LoadQueue();
queue.on("progress", handleProgress, this);
queue.on("complete", handleComplete, this);
queue.loadManifest([
{id: 'img1', src: 'https://images.unsplash.com/photo-1530379062299-a950185fdf6c?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=191d8c6bd6adc2e6b889bcde1731313c&auto=format&fit=crop&w=3000&q=100'},
{id: 'img2', src: 'https://images.unsplash.com/photo-1530758984765-9fa189425c28?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=f672f00aa31f1d63bf13e3af6d0e7aa8&auto=format&fit=crop&w=2500&q=100'},
{id: 'img3', src: 'https://images.unsplash.com/photo-1530750463537-af1c747d8d73?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=7e6252a08cf41d9b0ed59f08b3456854&auto=format&fit=crop&w=3000&q=100'}
]);
queue.load();
};
Now, time for the function which will be run when there is an update to the progress, handleProgress. We will take the event as an argument and grab the progress and multiply it by a hundred in order to get the progress in percent, round it down to an integer, then present this in the <span id=”loading-percent”></span> element we created earlier.
const handleProgress = (e) => {
let percent = Math.round(e.progress * 100);
document.getElementById('loading-percent').innerHTML = percent;
};
Finally, handleComplete:
const handleComplete = () => {
document.getElementById('preload-container').innerHTML = '<h1>COMPLETE!</h1>';
};
All the final JavaScript:
const loadAssets = () => {
document.getElementById('preload-container').innerHTML = '<h1>LOADING... <span id="loading-percent">' + 0 + '</span>%</h1>';
const queue = new createjs.LoadQueue();
queue.on("progress", handleProgress, this);
queue.on("complete", handleComplete, this);
queue.loadManifest([
{id: 'img1', src: 'https://images.unsplash.com/photo-1530379062299-a950185fdf6c?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=191d8c6bd6adc2e6b889bcde1731313c&auto=format&fit=crop&w=3000&q=100'},
{id: 'img2', src: 'https://images.unsplash.com/photo-1530758984765-9fa189425c28?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=f672f00aa31f1d63bf13e3af6d0e7aa8&auto=format&fit=crop&w=2500&q=100'},
{id: 'img3', src: 'https://images.unsplash.com/photo-1530750463537-af1c747d8d73?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=7e6252a08cf41d9b0ed59f08b3456854&auto=format&fit=crop&w=3000&q=100'}
]);
queue.load();
};
const handleProgress = (e) => {
let percent = Math.round(e.progress * 100);
document.getElementById('loading-percent').innerHTML = percent;
};
const handleComplete = () => {
document.getElementById('preload-container').innerHTML = '<h1>COMPLETE!</h1>';
};
Try it out for yourself with this CodePen:
See the Pen gKNYrY by Andreas Wik (@andreaswik) on CodePen.
We banged that one out, guys. On to the next one!