Extract GPS Location And Other EXIF Data From Photos Using JavaScript
July 15, 2018 by Andreas Wik
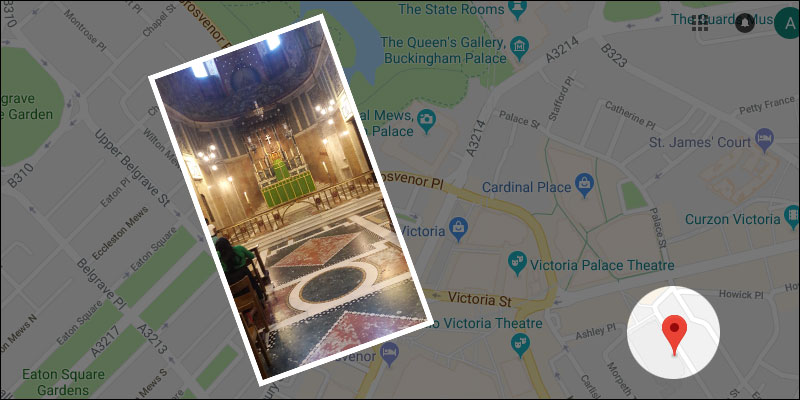
As I’m sure you know, or at least can imagine, photos often contain a lot of meta data “under the hood”. This is called EXIF data and contains info such as the device maker (e.g. Samsung), device model, date and time the photo was taken, GPS location data, and a lot of other stuff.
With exif.js we can easily use JavaScript to extract this data. Check out the example we’ll build.
Go ahead and download exif.js it or just grab it from a CDN (which I’ll do here).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Extract EXIF Data</title>
</head>
<body>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/exif-js/2.3.0/exif.min.js"></script>
<script>
// Our code goes here
</script>
</body>
</html>
Add a photo to the page along with a click event handler. To capture the data, we simply pass the image to the EXIF.getData function and store the data in our myData object. We can then just log myData.exifdata in the console to see all of the data available:
<img src="cathedral.jpg" id="the-img" style="max-width: 200px;"><br>
<p id="pic-info"></p>
<p id="map-link"></p>
// Our code goes here
document.getElementById("the-img").onclick = function() {
EXIF.getData(this, function() {
myData = this;
console.log(myData.exifdata);
});
}
In the console you’ll see something like this:
Let’s grab the device maker, model, as well as the date and time the photo was taken and print it out on the page.
document.getElementById('pic-info').innerHTML = 'This photo was taken on ' + myData.exifdata.DateTime + ' with a ' + myData.exifdata.Make + ' ' + myData.exifdata.Model;
To make it even more creepy, we can use the GPS data to work out where the photo was taken. It takes a bit of work to transfer the data we get into simple latitude and longitude values.
We can use myData.exifdata.GPSLatitude and myData.exifdata.GPSLongitude arrays (3 values in each: degree, minute, second), and also GPSLatitudeRef and GPSLongitudeRef to get latitude and longitude decimal values, which is what we need to find a location on Google Maps, for example.
Let’s write a function which will take the degrees, minutes, seconds and direction (E / W N / S) and turn it into a decimal number:
function ConvertDMSToDD(degrees, minutes, seconds, direction) {
var dd = degrees + (minutes/60) + (seconds/3600);
if (direction == "S" || direction == "W") {
dd = dd * -1;
}
return dd;
}
Okay, let’s wrap it up. We’ll call the function twice – once to get the Latitude and once to get the Longitude. Once we got the values, we’ll generate a link to Google Maps which will show the location.
// Calculate latitude decimal
var latDegree = myData.exifdata.GPSLatitude[0].numerator;
var latMinute = myData.exifdata.GPSLatitude[1].numerator;
var latSecond = myData.exifdata.GPSLatitude[2].numerator;
var latDirection = myData.exifdata.GPSLatitudeRef;
var latFinal = ConvertDMSToDD(latDegree, latMinute, latSecond, latDirection);
console.log(latFinal);
// Calculate longitude decimal
var lonDegree = myData.exifdata.GPSLongitude[0].numerator;
var lonMinute = myData.exifdata.GPSLongitude[1].numerator;
var lonSecond = myData.exifdata.GPSLongitude[2].numerator;
var lonDirection = myData.exifdata.GPSLongitudeRef;
var lonFinal = ConvertDMSToDD(lonDegree, lonMinute, lonSecond, lonDirection);
console.log(lonFinal);
// Create Google Maps link for the location
document.getElementById('map-link').innerHTML = '<a href="http://www.google.com/maps/place/'+latFinal+','+lonFinal+'" target="_blank">Google Maps</a>';
Stop Your Device From Storing The Location When Taking Photos
This stuff can feel pretty creepy, and rightfully so. Luckily, Facebook and most of the other big services remove EXIF data from the photos when you upload them. So no, anybody can’t just download photos from your Facebook profile and see all of these details.
To be on the safe side, though, you might just want to make sure your location isn’t saved at all, ever, to your photos. I googled for you, you’re welcome:
See you later, don’t be a creep!