Adjust Textarea Height While Typing To Fit Content With JavaScript
September 4, 2020 by Andreas Wik
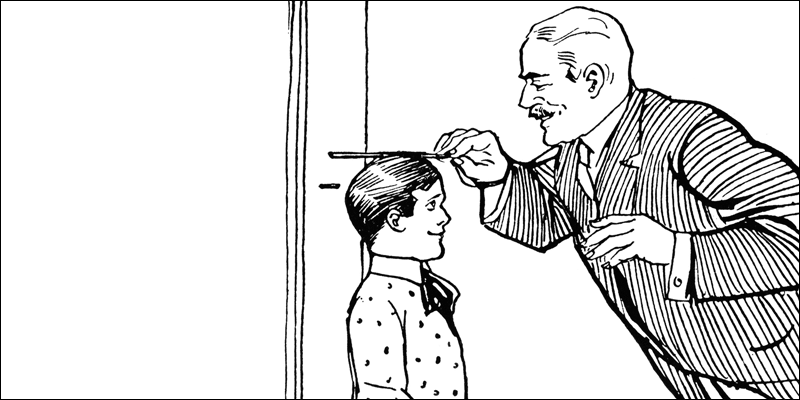
With a little bit of JavaScript we can adjust the height of a textarea while the user is typing to fit the text content.
Create a textarea on the page:
<textarea id="txt"></textarea>
With JavaScript, first select the element and add an event listener to it:
const textarea = document.getElementById("txt");
textarea.addEventListener("input", function (e) {
// Do stuff when the user types
});
The textarea‘s scrollHeight is what we want to use for height. So, in the event listener’s callback function, first set the textarea height to auto, then set it to the scrollHeight:
this.style.height = "auto";
this.style.height = this.scrollHeight + "px";
Final JavaScript:
const textarea = document.getElementById("txt");
textarea.addEventListener("input", function (e) {
this.style.height = "auto";
this.style.height = this.scrollHeight + "px";
});
In case you want to remove the scrollbar and the resize handle you can do that with the following CSS:
textarea {
resize: none;
overflow: hidden;
}
Playground, try it out:
See the Pen
Expand textarea height to fit content by Andreas Wik (@andreaswik)
on CodePen.