Allow Only Numbers In Input Field With JavaScript
February 13, 2023 by Andreas Wik
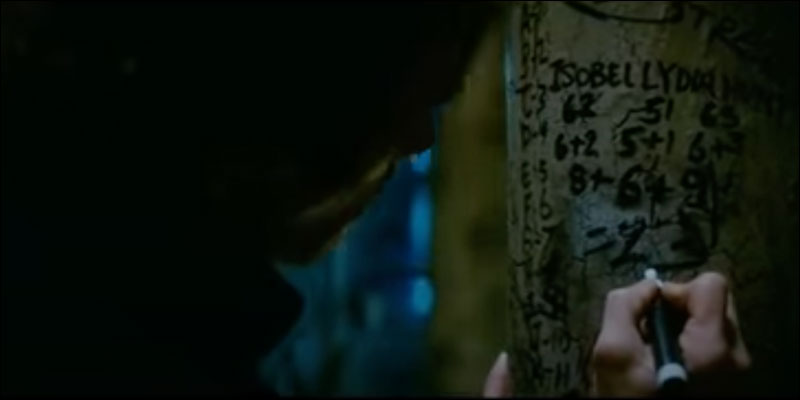
Let’s look at how we can force users to type only digits into an input field with some JavaScript.
Sure, you can use type=”number” for your input field, but there may be situations where it could be useful to use JavaScript to only allow certain characters or digits to be typed into a field. In this example I’m setting the input field type to text.
First of all, let’s add an input field to our page.
<input id="numbers-only" type="text" placeholder="Numbers Only">
Next, it’s time for the JavaScript.
Select the input field:
const inputField = document.querySelector('#numbers-only');
Let’s then create an event handler for the onkeydown event.
inputField.onkeydown = function(event) {
};
In this case, we will only allow digits to go through. We can get the key pressed via event.key. Using this we can use isNaN (a function which returns true if the value passed is not a number and false otherwise) to determine whether or not it’s a number. If it’s not a number, we call event.preventDefault() which will basically stop the character from being added to the input field’s value.
inputField.onkeydown = function(event) {
// Only allow if the e.key
if(isNaN(event.key)) {
event.preventDefault();
}
};
We also want the user to be able to hit backspace to remove digits, so let’s add in another condition for that.
inputField.onkeydown = function(event) {
// Only allow if the e.key value is a number or if it's 'Backspace'
if(isNaN(event.key) && event.key !== 'Backspace') {
event.preventDefault();
}
};
Here is a CodePen you can play around with:
See the Pen Untitled by Andreas Wik (@andreaswik) on CodePen.
Cheers!