Check If Element Is Inside Of The Viewport With JavaScript
August 2, 2019 by Andreas Wik
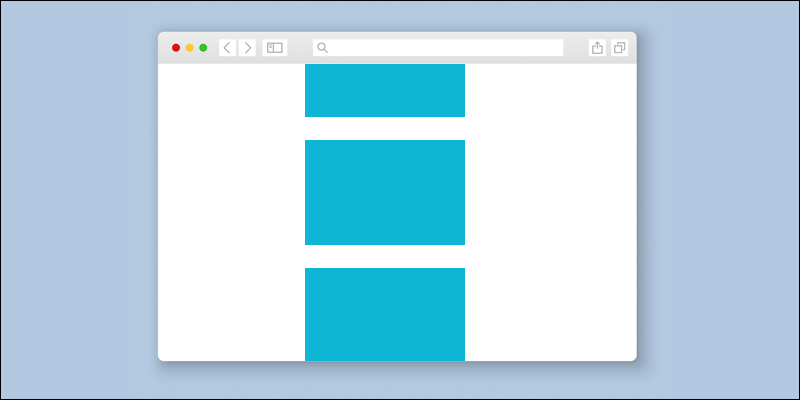
Let’s have a look at how we can check if a certain element on the page is partially or fully inside of the viewport. This could be used for a lot of things, a classic use case would be lazy loading images. Another use case could be to trigger an animation when the element comes into the viewport.
First we need to find out how far from the edges of the viewport the element is currently positioned by using JavaScript’s function getBoundingClientRect().
To find out if the whole element is inside of the viewport we can simply check if the top and left value is bigger or equal to 0, that the right value is less or equal to the viewport height ie window.innerWidth and that the bottom value is less or equal to window.innerHeight.
var myElement = document.getElementById('my-element');
var bounding = myElement.getBoundingClientRect();
if (bounding.top >= 0 && bounding.left >= 0 && bounding.right <= window.innerWidth && bounding.bottom <= window.innerHeight) {
console.log('Element is in the viewport!');
} else {
console.log('Element is NOT in the viewport!');
}
Something to keep in mind is that window.innerWidth and window.innerHeight doesn’t work in all browsers. To make sure this doesn’t break in those browsers, let’s use document.documentElement.clientWidth and document.documentElement.clientHeight.
So instead of:
bounding.right <= window.innerWidth
We write the following, which falls back to document.documentElement.clientWidth if window.innerWidth is not supported:
bounding.right <= (window.innerWidth || document.documentElement.clientWidth)
And so the final code to check if the whole element is inside of the viewport is:
var myElement = document.getElementById('my-element');
var bounding = myElement.getBoundingClientRect();
function elementInViewport() {
var bounding = myElement.getBoundingClientRect();
if (bounding.top >= 0 && bounding.left >= 0 && bounding.right <= (window.innerWidth || document.documentElement.clientWidth) && bounding.bottom <= (window.innerHeight || document.documentElement.clientHeight)) {
console.log('Element is in the viewport!');
} else {
console.log('Element is NOT in the viewport!');
}
}
See the Pen Check if the whole element is inside of viewport by Andreas Wik (@andreaswik) on CodePen.
Check if part of element is in the viewport
In most cases, I think it makes more sense to find out if any part of the element is inside of the viewport, especially in the case of lazy loading images. So let’s do that.
Let’s grab the width and height of our element using myElement.offsetWidth and myElement.offsetHeight.
var myElement = document.getElementById('my-element');
var bounding = myElement.getBoundingClientRect();
var myElementHeight = myElement.offsetHeight;
var myElementWidth = myElement.offsetWidth;
function elementInViewport() {
var bounding = myElement.getBoundingClientRect();
if (bounding.top >= -myElementHeight
&& bounding.left >= -myElementWidth
&& bounding.right <= (window.innerWidth || document.documentElement.clientWidth) + myElementWidth
&& bounding.bottom <= (window.innerHeight || document.documentElement.clientHeight) + myElementHeight) {
console.log('Element is in the viewport!');
} else {
console.log('Element is NOT in the viewport!');
}
}
Try it in the CodePen below – scroll up, down and sideways and hit the check button to see if part of the element is in the viewport:
See the Pen Check if part of element is inside of the viewport by Andreas Wik (@andreaswik) on CodePen.