Collect Daily And Historical Stock Prices
June 21, 2018 by Andreas Wik
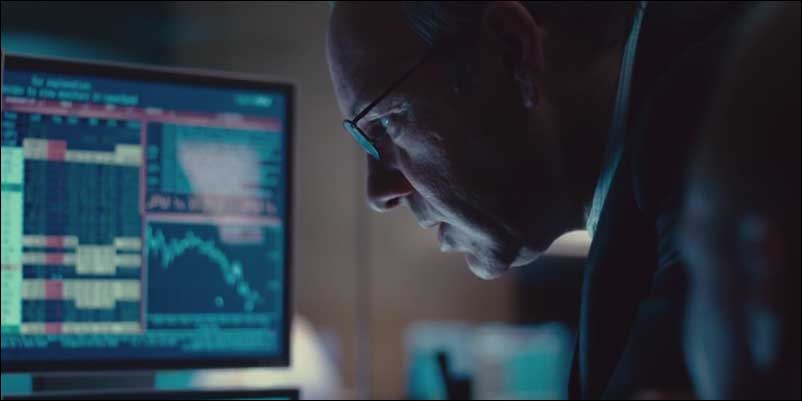
Not too long ago I was really struggling to find a good, free API to collect stock prices for a little app I was building. Many people had been recommending the Yahoo Finance API for a few years, but this was now deprecated, although some still used it with some sort of hacky workaround. I read on different forums that Google used to have a good one, but that had been abandoned as well.
I was starting to think I would have to scrape the data off of some finance site, but oh my, the time it would take is ridiculous compared to a few API calls.
Then I ran into this amazing API: AlphaVantage – a completely free, robust finance API which provides daily price points and other data about both stocks, crypto currencies and currency exchange rates.
This is the API I used to create CashPuppet.
It’s really quick and easy to get started. Here are two links you can check out just to see what the output looks like. First one gets the latest prices for the Microsoft (MSFT is the stock symbol) stock and the second one for Apple (APPL):
https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=MSFT&apikey=demo
https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=APPL&apikey=demo
This will get you the stock price for the last 100 market days. Four different prices for each per date – the price when the market opened, when it closed, the lowest of the day, and the highest of the day.
You can choose, however, to get all the price points they’ve got (going back to the year of 2000) by setting the parameter outputsize to full. By default it’s set to compact.
Like so:
https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=APPL&outputsize=full&apikey=demo
Remember to replace demo with your own API key.
A quick way to find a symbol for a stock is to just google it and you’ll be presented with some info. “McDonalds stock” gives you this:
As you can see, the stock symbol is “MCD” which is what you’ll use when calling the AlphaVantage API.
Let’s code
With a bit of JavaScript (using jQuery in this example) we’ll grab the price of McDonald’s (MCD) stock for the past 100 market days.
$.ajax({
type: 'GET',
url: 'https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=MCD&apikey=YOUR-API-KEY',
dataType: 'json',
beforeSend:function() {
console.log('Sending request...');
},
error: function(jqXHR, textStatus, errorThrown) {
console.log('Fail: ' + errorThrown);
},
success: function(data) {
var i = 0;
$.each(data, function(objectTitle, objects) {
if(i >= 1) {
$.each(objects, function(objectKey, objectValue) {
console.log('');
console.log(objectKey);
console.log('OPEN: ' + objectValue['1. open']);
console.log('HIGH: ' + objectValue['2. high']);
console.log('LOW: ' + objectValue['3. low']);
console.log('CLOSE: ' + objectValue['4. close']);
});
}
i++;
});
}
});
Same thing with PHP:
$curl_str = 'https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=MCD&apikey=YOUR-API-KEY';
$curl = curl_init($curl_str);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($curl);
$result = json_decode($result, true);
foreach ($result['Time Series (Daily)'] as $key => $value) {
echo $key . '<br>';
echo 'OPEN: ' . $value['1. open'] . '<br>';
echo 'HIGH: ' . $value['2. high'] . '<br>';
echo 'LOW: ' . $value['3. low'] . '<br>';
echo 'CLOSE: ' . $value['4. close'] . '<br><br>';
}
If you build something cool using the AlphaVantage API I’d love to check it out – let me know!