Cut Off Text Nicely With text-overflow
November 11, 2017 by Andreas Wik
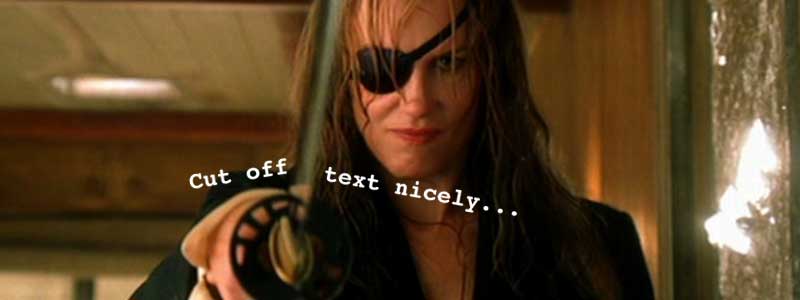
I was working on this page that had a list of company summaries, where each item should be a single line. Needless to say, some longer summaries won’t fit on one line. The solution was to simply add white-space: nowrap; and overflow: hidden; so that if the text is too long, it’s cut off and the rest is hidden.
ul li {
white-space: nowrap;
overflow: hidden;
}
Output:
Great, problem solved!
Right?
Yeah, but… It really doesn’t look great, does it? Looks kinda broken when you cut off text just like that. Maybe I could use Javascript to find out where the text is cut off and add three dots ” … ” or something. Hmm…
Setting text-overflow to ellipsis achieves exactly this. It adds … to the position where it’s cut off.
Quick fix!
ul li {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
Output:
Browser Support
While the support for text-overflow: ellipsis is good, as caniuse.com states, there are issues in some browsers when using this on specific form elements.
The only values that seem to work well are clip (which just cuts off the text, giving the same result as the first example not using text-overflow at all) and ellipsis. There are a few more options, but the browser support for these varies, to say the least.
You can read more about them here.
Alternative Javascript solution
While this worked great for my specific purpose, there are other cases where it might not. I mean, you might not want to be looking to keep it on a single line, but on multiple lines and cut the text after a certain number of characters instead.
Well in that case, here is a little Javascript function which cuts off the string you pass it and return the first X number of characters.
function shorten(text, maxLength) {
// First check if the text is too long
if (text.length > maxLength) {
// Shorten text to preferred length and add ... to the end of it
text = text.substr(0, maxLength-3) + "...";
}
return text;
}
So let’s use this function to get the first 100 characters of a string and print it out on the page:
<p></p>
$(document).ready(function() {
// Original text
var summaryLong = "SoundCloud is an online audio distribution platform based in Berlin, Germany, that enables its users to upload, record, promote, and share their originally-created sounds.";
// Shorten the text
var summaryShort = shorten(summaryLong, 100);
// Add the short new text to element
$('p').text(summaryShort);
});
function shorten(text, maxLength) {
// First check if the text is too long
if (text.length > maxLength) {
// Shorten text to preferred length and add ... to the end of it
text = text.substr(0, maxLength-3) + "...";
}
return text;
}
Output:
Handy.