Dynamically Load And Apply Fonts With JavaScript
July 10, 2020 by Andreas Wik
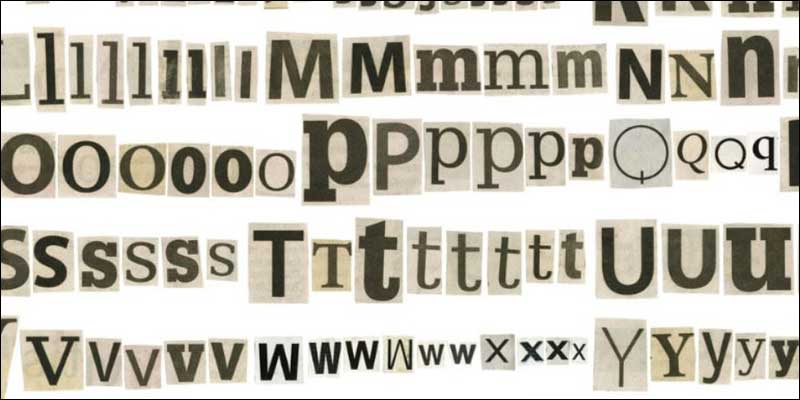
The CSS Font Loading API lets you load fonts dynamically with JavaScript only if and when you need them.
Let’s say we’re building a little editor where the user can pick from 20 different fonts for their text. Rather than having every user load all 20 fonts, which is what would happen using the traditional CSS @font-face method, we can load a font with JavaScript only if a user requests it.
The first step is to define a FontFace object for the font. Pass on two parameters, the first being the a name and the second being the path to the font.
// Your font goes here
const bangersFont = new FontFace('Bangers Regular', 'url(Bangers-Regular.ttf)');
You can then go ahead and load the font only when and if you need it.
bangersFont.load().then(function(loadedFont) {
document.fonts.add(loadedFont)
text.style.fontFamily = '"Bangers Regular"';
}).catch(function(error) {
console.log('Failed to load font: ' + error)
})
Apply it to an element.
text.style.fontFamily = '"Bangers Regular"';
Complete code with the loading of the font put in a function. When the user clicks the button, the font is loaded and applied.
<button id="btn" type="button">Load font</button>
<h1 id="font-presentation">Nice font here</h1>
// Button element
const btn = document.getElementById('btn')
btn.onclick = loadFont
// H1 element
const text = document.getElementById('font-presentation')
// Your font goes here
const bangersFont = new FontFace('Bangers Regular', 'url(Bangers-Regular.ttf)');
// Function which loads the font and applies it to the H1
function loadFont() {
console.log('Loading font...')
bangersFont.load().then(function(loadedFont) {
document.fonts.add(loadedFont)
text.style.fontFamily = '"Bangers Regular"';
}).catch(function(error) {
console.log('Failed to load font: ' + error)
})
}
You can read more on MDN about the CSS Font Loading API.
Have fun out there, take care!