Loop Through Object In JavaScript
May 8, 2020 by Andreas Wik
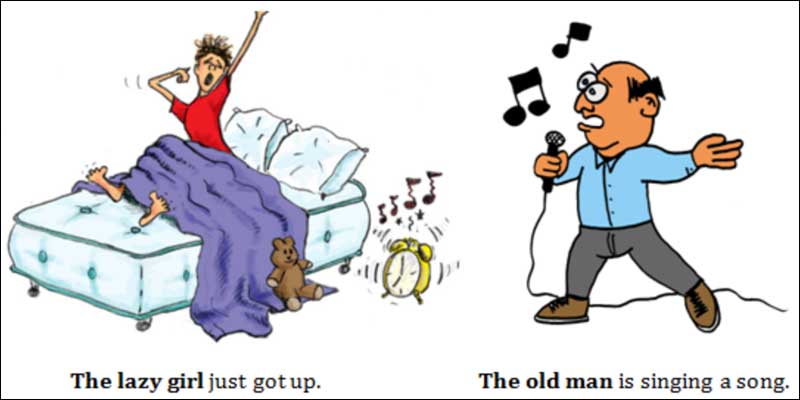
Three ways to loop through an object in JavaScript. Get both keys and values, only keys, or only values.
Get both keys and values
To get both the keys and values, Object.entries will get the job done. It returns an array of an array, where each child array holds the key in the first slot and the value in the second.
We can then loop through the returned array and do something with the key and value, see below:
const population = {
Italy: 10,
Zimbabwe: 14,
Chile: 19
}
// Get array containing keys and values from Object.entries
const populationEntries = Object.entries(population)
// Loop through the returned array
for(const populationEntry of populationEntries) {
console.log(`${populationEntry[0]}: ${populationEntry[1]}`)
}
/*
Console log:
Italy: 10
Zimbabwe: 14
Chile: 19
*/
Let’s make it a little nicer by destructuring the array:
// Same thing with array destructuring
for(const [country, population] of populationEntries) {
console.log(`${country}: ${population}`)
}
Get the keys
If only the keys are of interest, we can use Object.keysΒ which returns an array containing the keys. Nice and simple.
const keys = Object.keys(population)
for(const key of keys) {
console.log(key)
}
/*
Console log:
Italy
Zimbabwe
Chile
*/
Get the values
Object.values returns an array containing the values:
const values = Object.values(population)
for(const value of values) {
console.log(value)
}
/*
Console log:
10
14
19
*/
Take care of yourself, God bless!