Loop Through All Posts In single.php Or Other Page – WordPress
June 30, 2023 by Andreas Wik
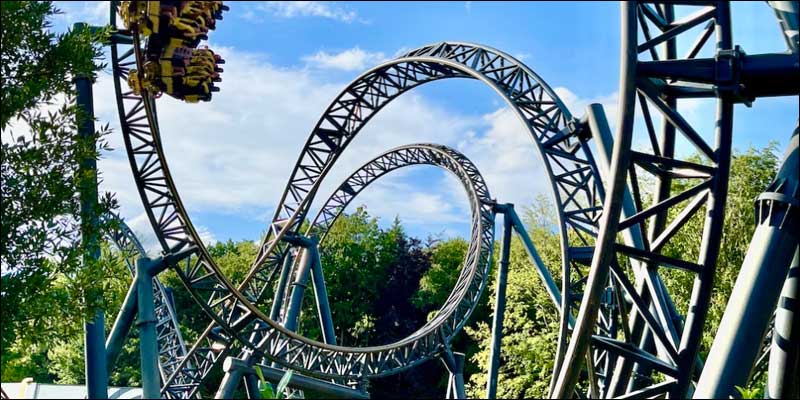
Looping through your posts in index.php is fairly straightforward. You may have something like this set up:
<?php
if(have_posts()) : while(have_posts()) : the_post();
?>
<div class="post">
<h3><?php the_title(); ?></h3>
<p><?php the_content(); ?></p>
</div>
<?php
endwhile;
endif;
?>
You may think that in single.php you should be able to do the same. Certainly looks like it. However, since single.php is designed to show a single post, that’s all you’ll get using the same loop – the current post.
In order to loop through all posts, you can make use of WP_Query, passing it a few arguments. In the example below we ask to retrieve only posts with the post type ‘posts’: ‘post_type’ => ‘post’. We also ask to get all posts with ‘posts_per_page’ => -1.
Then we can simply loop through them, printing out the title, content and/or whatever else you want to show, perhaps just the excerpt and the featured image.
<?php
$args = array(
'post_type' => 'post',
'posts_per_page' => -1,
);
$posts_query = new WP_Query($args);
if ($posts_query->have_posts()) {
while ($posts_query->have_posts()) {
$posts_query->the_post();
?>
<!-- Show post -->
<div class="post">
<h3><?php the_title(); ?></h3>
<p><?php the_content(); ?></p>
</div>
<?php
}
}
wp_reset_postdata();
?>
Hope it helps!