Make Your Form JavaScript Validation Errors Accessible For Screen Readers
June 30, 2018 by Andreas Wik
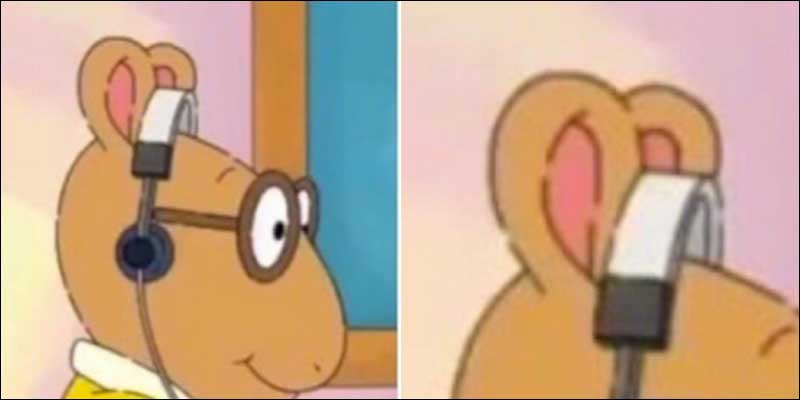
Accessibility is important, and here follows an easy way to make your JavaScript errors accessible for screen readers when, for example, validating forms.
Both Windows and Mac OS have built-in voice over screen readers to help people with disabilities. Try it for yourself, turn it on/off using Cmd + F5 for Mac or Windows Key + Enter for Windows. It reads what’s on the page and tries to understand how to fill in forms etc.
In this little exercise we will set up a basic form, validate it with JavaScript, and make sure that the screen readers reads out the error for the user.
Markup:
<div id="container">
<h1>Newsletter Signup</h1>
<form>
<div id="error"></div>
<input id="first-name" type="text" placeholder="First Name"><br>
<input id="email" type="text" placeholder="Email"><br>
<button type="button" onclick="join();">Join</button>
</form>
</div>
JavaScript – the validate function:
function join() {
let firstname = document.getElementById('first-name').value;
let email = document.getElementById('email').value;
if(firstname.length < 1) {
document.getElementById('error').innerHTML = 'Please enter your first name';
document.getElementById('first-name').focus();
}
else if(email.length < 1) {
document.getElementById('error').innerHTML = 'Please enter your email';
document.getElementById('email').focus();
}
else {
// Success - save the user data
document.getElementById('container').innerHTML = 'Thanks!';
}
}
Turn on the screen reader and click “Join” but leave the email field empty. It will trigger an error:
See the Pen GGzZEO by Andreas Wik (@andreaswik) on CodePen.
Problem: The screen reader doesn’t notice the change of the error div, and so the blind user wouldn’t know there is an error.
The Solution
Fortunately there’s an attribute called aria-live. Setting this value to assertive will make sure the screen reader picks up on changes in the element and will read the new content out.
So let’s add aria-live=”assertive” to our error element:
<div id="error" aria-live="assertive"></div>
Try the same thing once again with this CodePen:
See the Pen LrJrjr by Andreas Wik (@andreaswik) on CodePen.
Now the screen reader actually reads out the error for the user – nice!
aria-live
The aria-live attribute can have three values: Off, Polite or Assertive.
Off is obviously doing what it says, the screen reader will never catch updates for this element. The polite setting is, according to the spec:
“Any updates made to this region should only be announced if the user is not currently doing anything. live=”polite” should be used in most situations involving live regions that present new information to users, such as updating news headlines.”
In our case we are using Assertive, and if your read the spec for it you’ll realize why:
“Any updates made to this region are important enough to be announced to the user as soon as possible, but it is not necessary to immediately interrupt the user. live=”assertive” must be used if there is information that a user must know about right away, for example, warning messages in a form that does validation on the fly.”
We are dealing with form errors and we definitely want to make sure the user is aware of them.
Final Notes On Accessibility
“Make sure the website is accessible” can sound like a daunting task if you haven’t had to deal with it before. However, most often it’s really quick stuff that needs to be done to tick that list off. It’s definitely worth taking a couple of hours to read up on general accessibility best practices.