Measure Your JavaScript Code Execution Time
July 24, 2018 by Andreas Wik
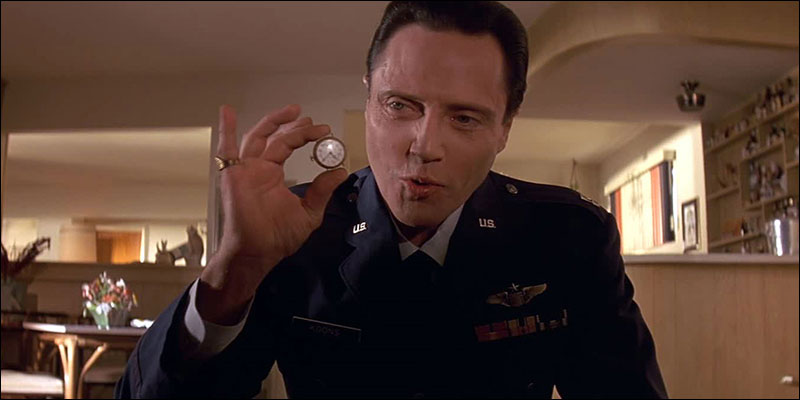
There are times when you want and need an easy way to measure JavaScript performance. With console.time() and console.timeEnd() you can easily log the execution execution time in milliseconds in the console.
Simply run console.time(“name of my timer”) when you want to start logging the time and console.timeEnd(“name of my timer”) when the code you wish to measure has finished executing.
Works just like a good ol’ stopwatch.
Let’s try it out with a for loop. The following code will log how long it takes to run a for loop ten million times:
var numba;
console.time('For Loop 10 Million');
// Loop through 10 million times
for(numba = 0; numba < 10000000; numba++) {
}
console.timeEnd('For Loop 10 Million');
Let’s do the same thing with an API call. The following code logs how long a call to the Google Books API takes:
$.ajax({
type: 'GET',
url: 'https://www.googleapis.com/books/v1/volumes?q=isbn:0747532699',
dataType: 'json',
beforeSend:function() {
console.time('Google Books API Call');
},
error: function(jqXHR, textStatus, errorThrown) {
console.log('API Call Failed: ' + errorThrown);
},
success: function(data) {
console.timeEnd('Google Books API Call');
}
});
Access The Result In Code
There is an alternative method you can use in case you need to have access to the result in your code. This is basically to get the current time in milliseconds before and after the execution using Date() and getTime().
Like so:
var startDate, msStart, endDate, msEnd, msExec, numba;
// Get start time
startDate = new Date();
msStart = startDate.getTime();
// Do something
for(numba = 0; numba < 10000000; numba++) {
}
// Get end time
endDate = new Date();
msEnd = endDate.getTime();
// End time minus start time to get execution time
msExec = msEnd - msStart;
console.log('It took: ' + msExec + ' milliseconds');
Fire up the console and head over to this page to run the code snippets above.
That’s all for now. Over and out.
/Koons