Order Array Of Objects By Property Value In JavaScript
May 22, 2020 by Andreas Wik
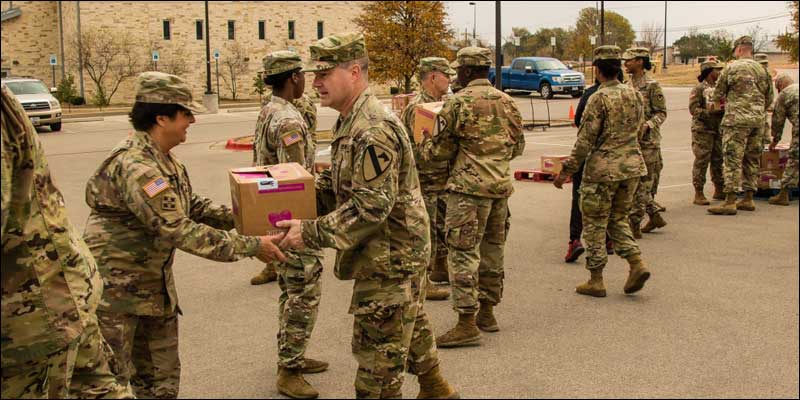
Imagine that you have an array of objects and want to order them depending on the value of a specific property in the objects.
With sort() we can do exactly this. In the example below I want to order the players by their score.
const players = [
{
nick: 'ChuckNoir',
location: 'US',
score: 154
}, {
nick: 'Livarios',
location: 'IT',
score: 732
}, {
nick: 'pippi',
location: 'SE',
score: 540
}
]
// ORDER:
// ChuckNoir 154
// Livarios 732
// pippi 540
Just running players.sort() isn’t gonna do what we want. We need to provide sort with a compare function. It will take two arguments (in this example called a and b) which represents two objects to compare. If the score property value of a is larger than that of b, then b will come before a in the array.
players.sort((a, b) => (a.score > b.score) ? 1 : -1)
// NEW ORDER:
// ChuckNoir 154
// pippi 540
// Livarios 732
To flip the order, going from high to low score, simply tweak the comparison to a.score < b.score.
players.sort((a, b) => (a.score < b.score) ? 1 : -1)
// NEW ORDER:
// Livarios 732
// pippi 540
// ChuckNoir 154
Ha de gott, kompis!