Switch Between Dark Theme And Light Theme With CSS And JavaScript
July 19, 2023 by Andreas Wik
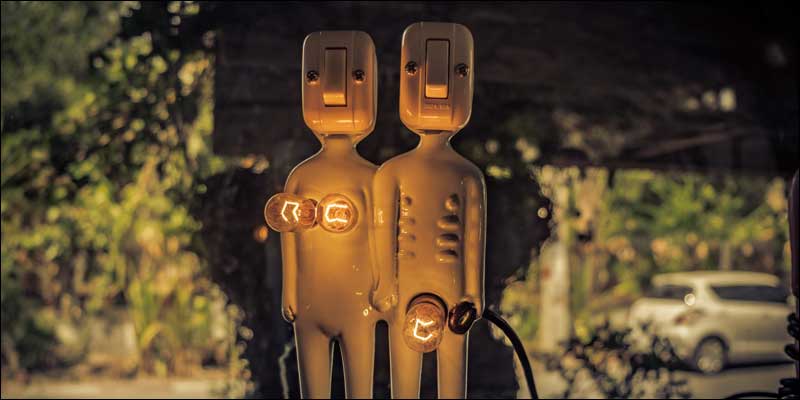
Using CSS variables we can quite easily create various color themes for websites and apps. The most popular way is to let users toggle between a light and a dark theme. Here’s one way to do just that.
We will create two 2 sets of variables in CSS for the colors for each theme. We will toggle data-theme on the html element so that when it changes, the values for the color variables change as well.
/* Light colors */
html {
--background-color-primary: #ffffff;
--background-color-secondary: #eeeeee;
--text-color: #333333;
}
/* Dark colors */
html[data-theme="dark"] {
--background-color-primary: #10162a;
--background-color-secondary: #151e39;
--text-color: #94a3b8;
}
Let’s use these variables to style a couple of elements.
body {
background-color: var(--background-color-primary);
}
p {
color: var(--text-color);
}
img {
border: 10px solid var(--background-color-secondary);
}
In the markup, why don’t we add a checkbox which the user can check or uncheck depending on whether they want the light or dark theme.
<input id="theme-switch" type="checkbox">
Now we also need a little bit of JavaScript so that when the state of the checkbox changes, we also change the value of data-theme.
const themeSwitch = document.querySelector('#theme-switch');
themeSwitch.addEventListener('change', () => {
if(themeSwitch.checked) {
document.documentElement.setAttribute('data-theme', 'dark');
} else {
document.documentElement.setAttribute('data-theme', 'light');
}
});
That is really all it takes to get it working, then you can expand with way more variables, of course, and style the checkbox nicely, add some transition to make the switch smoother.
Get user’s preferred theme browser setting
There are a couple of ways to find out whether the user prefers a light or dark theme, depending on their browser settings. Check out my article here: Check if user has browser theme preference set to light or dark with JavaScript.
Below is a CodePen you can check out. Hit the little sun or moon icon in the header to switch between the light and the dark theme.
See the Pen Untitled by Andreas Wik (@andreaswik) on CodePen.