Turn Headline Into URL Friendly Slug With PHP
November 11, 2017 by Andreas Wik
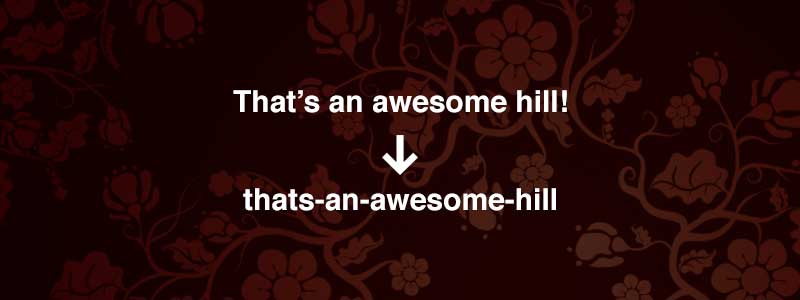
This is a short one. This little code snippet will turn a text into a URL friendly slug, similar to what WordPress does.
Here’s the function:
function slugify($string) {
// Replaces all spaces with hyphens
$string = str_replace(' ', '-', $string);
// Replace accent characters
$fromto = array('à'=>'a', 'À'=>'a', 'â'=>'a', 'Â'=>'a', 'á'=>'a', 'Á'=>'a', 'å'=>'a', 'Å'=>'a', 'ä'=>'a', 'Ä'=>'a', 'è'=>'e', 'È'=>'e', 'é'=>'e', 'É'=>'e', 'ê'=>'e', 'Ê'=>'e', 'ì'=>'i', 'Ì'=>'i', 'í'=>'i', 'Í'=>'i', 'ï'=>'i', 'Ï'=>'i', 'î'=>'i', 'Î'=>'i', 'ò'=>'o', 'Ò'=>'o', 'ó'=>'o', 'Ó'=>'o', 'ö'=>'o', 'Ö'=>'o', 'ô'=>'o', 'Ô'=>'o', 'ù'=>'u', 'Ù'=>'u', 'ú'=>'u', 'Ú'=>'u', 'ü'=>'u', 'Ü'=>'u', 'û'=>'u', 'Û'=>'u', 'ñ'=>'n', 'ç'=>'c', '·'=>'-', '/'=>'-', '_'=>'-', ','=>'-', ':'=>'-', ';'=>'-');
$string = strtr($string, $fromto);
// Make lowercase
$string = strtolower($string);
// Remove special chars
$string = preg_replace('/[^A-Za-z0-9\-]/', '', $string);
// Replaces multiple hyphens with single one
return preg_replace('/-+/', '-', $string);
}
So to use it, we’ll just go ahead and call the function with our string.
$headline = "That's an amazing hill!!!";
echo $headline . '<br>';
$slug = slugify($headline);
echo $slug;
Output:
That’s an amazing hill!!! thats-an-amazing-hill