Using Local Storage With JavaScript – The Database Built Into Your Browser
November 17, 2018 by Andreas Wik
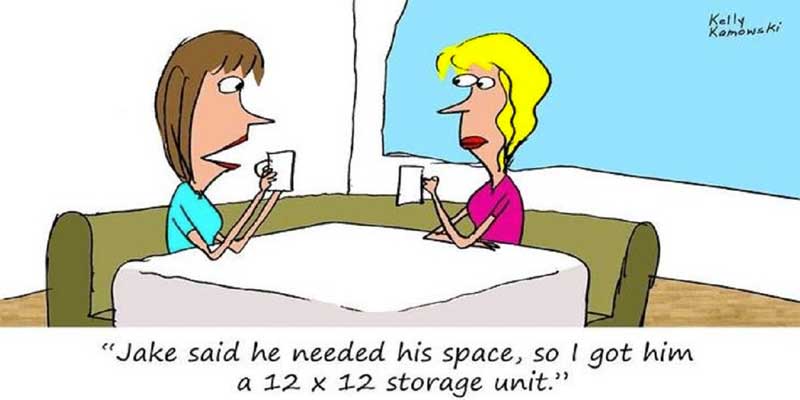
Local Storage is a storage in your browser where you can easily save data in key value pairs. It’s a really handy alternative to cookies as you have a lot more storage to work with (around 5MB compared to the few KB’s you can use for cookies, although it does depend on the browser at the end of the day, can be less, can be more).
Only String Values And No Expiry Time
A couple of things worth noting…
All your local storage values will be saved and retrieved as strings, so in order to work with numbers or arrays etc. you’ll have to perform some operations, for example encode and decode your data using JSON.
There is no way to set when the data should expire and be wiped out, this is entirely up to the browser settings or whenever the user clears their local storage manually.
Store And Fetch Data
The data entries in the local storage are saved like this:
KEY | VALUE
KEY | VALUE
KEY | VALUE
So to store something using JavaScript we use localStorage.setItem(key, value) where the key is the unique identifier for this entry and that you use to retrieving the data, and the value being whatever you want to store – a name, a counter.
Let’s store your name:
localStorage.setItem(“name”, “Andreas”);
To fetch this entry we use localStorage.getItem(key), like so:
localStoraget.getItem(“name”);
Worth to note is that if there is nothing in the storage with that key, then null is returned.
Working With Numbers
As mentioned earlier, a value stored in local storage is always treated as a string. So even if you would store an integer, localStorage(“counter”, 5), local storage will treat it as if you wrote localStorage(“counter”, “5”).
For example:
let number;
localStorage.setItem("number", 5);
number = localStorage.getItem("number");
if(number) {
console.log(number + 2);
}
Console output:
52
What we would want here is the output to be 7, right? But it will be 52 since the number variable is treated as a String.
So in order to work with it as an integer we must turn it into one using parseInt():
if(number) {
number = parseInt(number);
console.log(number + 2);
}
Console output:
7
Excellent.
Working With JSON Data
To work with a bit more advanced data we can use JSON and take advantage of JavaScript’s JSON.stringify() when storing and JSON.parse() when retrieving.
Let’s store an array of pets, each of them having a name, type (of animal), and mood.
// Fetch entry "pets" from local storage
let pets = localStorage.getItem('pets');
// Check so pets is not null
if (pets) {
document.body.innerHTML = "There is data in local storage<br><br>";
// There is data in the local storage, let's parse it and turn it into JSON
pets = JSON.parse(pets);
} else {
// pets is null, so let's generate the pets array and store it
document.body.innerHTML = "No data found in local storage - generating...<br><br>";
// No data in local storage, so create array of pets, each with some properties
pets = [{
name: 'Liz',
animal: 'turtle',
mood: 'pretty happy'
}, {
name: 'Alfred',
animal: 'worm',
mood: 'depressed'
}, {
name: 'Zoey',
animal: 'antelope',
mood: 'furious'
}];
// Turn the pets array into a string
const jsonPets = JSON.stringify(pets);
// Store it
localStorage.setItem('pets', jsonPets);
}
// Loop through the pets and print on the page and in the console
pets.forEach(function(pet) {
document.body.innerHTML += pet.name + " is a " + pet.mood + " " + pet.animal + "<br><br>";
console.log(pet.name + " is a " + pet.mood + " " + pet.animal);
});
Delete Data
Wiping an entry in the storage is as simple as localStorage.clear(key);.
localStorage.clear(pets);
Today was a good day.