Using Media Queries In JavaScript
August 4, 2020 by Andreas Wik
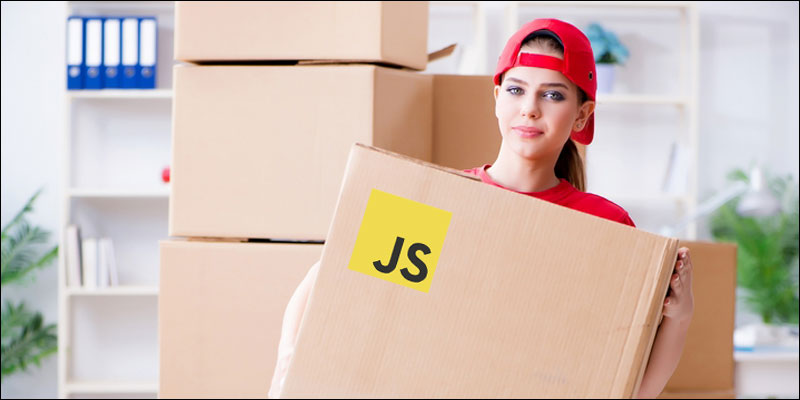
I bet you use media queries in CSS all the time, like:
@media only screen and (max-width: 600px) {
#footer {
display: block;
}
}
This will apply some styles for screens up to 600px wide.
We can actually also do this in a very similar fashion in JavaScript, using the built in window.matchMedia method. Call window.matchMedia(rule) with rule being, for example, “(max-width: 600px)”.
window.matchMedia("(max-width: 600px)")
We could then access the matches property on the returned object to see if it matches and, depending on the result, do something like adding a class to a DOM element.
const container = document.getElementById('content')
const small = window.matchMedia("(max-width: 600px)").matches
if (small) {
container.classList.add("small")
} else {
container.classList.add("large")
}
It’s also possible to keep track of when a media query match changes. Building on the example from above, I want my updateWindowSize function to run every time the media query matching result changes, e.g. window resizing in this case.
function updateWindowSize() {
const small = window.matchMedia("(max-width: 600px)").matches
if (small) {
container.classList.remove("large")
container.classList.add("small")
container.innerHTML = "SMALL<br>WINDOW"
} else {
container.classList.remove("small")
container.classList.add("large")
container.innerHTML = "LARGE<br>WINDOW"
}
}
window.matchMedia("(max-width: 600px)").addListener(updateWindowSize)
Playground:
Wickeeed.