Write To And Append To File With Node.js
August 10, 2023 by Andreas Wik
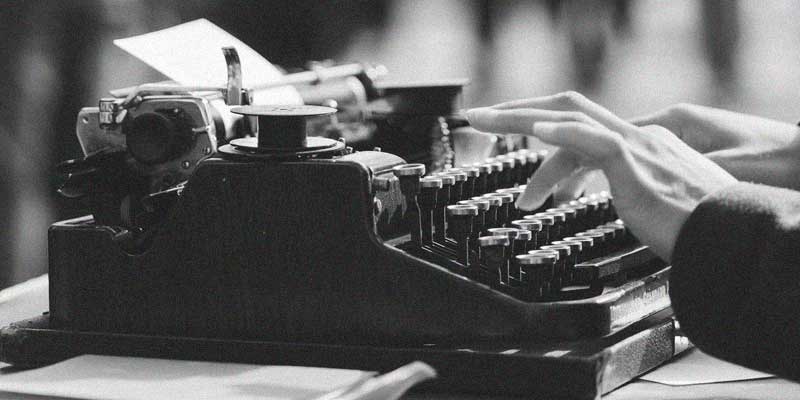
It’s quick and easy to write to a file with Node.js.
First, import the fs (file system) module.
const fs = require('fs');
Let’s create some data that we can write to a JSON file.
const people = [
{
name: 'Kim',
age: 27,
occupation: 'Veterinarian'
}, {
name: 'Alex',
age: 20,
occupation: 'Video store clerk'
}
];
Write to file
To create a new file and write to it, we use fs.writeFile and pass in the filename, the content and an error handler.
fs.writeFile('people.json', JSON.stringify(people), error => {
if (error) {
console.error(error);
}
});
That’s it, you should now see a people.json file with the content in the folder where your JS file lives.
Append to file
In order to append content to an already existing file, we simply use fs.appendFile instead, again passing in the filename, content and an error handler.
fs.appendFile('people.json', people, error => {
if (error) {
console.error(error);
}
});
If you open the file you wrote to now you should see the new data at the end of it.
Auf Wiedersehen.